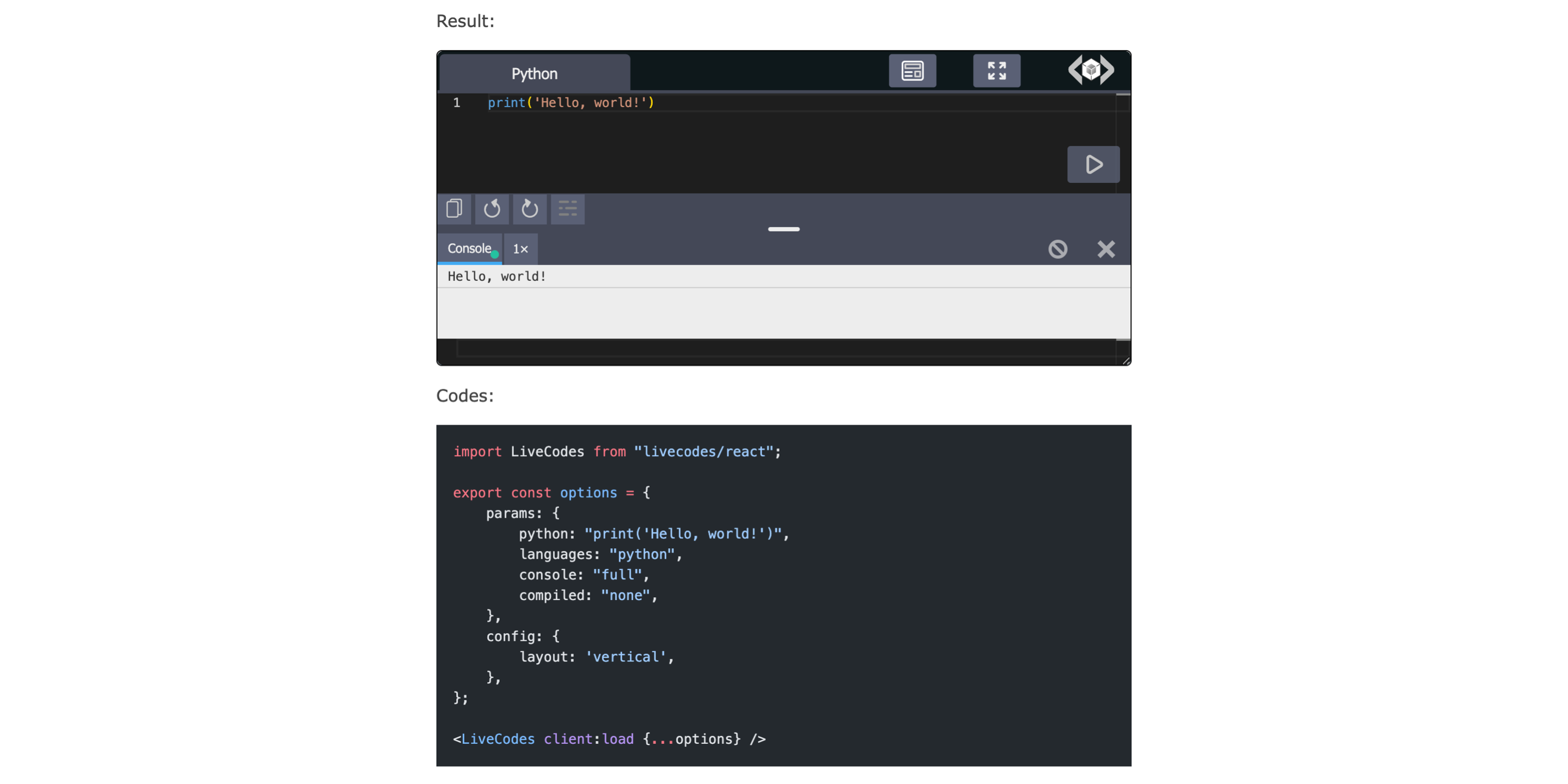
Run Python Code Embedded in Web
Introduction
Running Python in web pages can be useful for several reasons:
- Interactive Learning: It allows users to learn and practice Python directly within the web page without needing to set up a local development environment.
- Demonstrations: It can be used to demonstrate Python code snippets and their outputs in real-time, making tutorials and documentation more interactive.
- Prototyping: Users can quickly prototype and test Python code within the browser.
- Engagement: Interactive content can increase user engagement on educational and technical blogs or websites.
This is typically achieved using tools like Runno or LiveCodes, which embed a Python runtime in the web page, allowing users to write, run, and see the output of Python code directly in their browser.
Tools for Embedding Python
Here are some tools for embedding Python code in web pages:
-
Runno: A tool that allows you to run Python code directly in the browser. It provides an interactive environment for executing Python scripts.
-
LiveCodes: A versatile tool that supports multiple languages, including Python. It allows you to embed code editors and run code snippets directly in the browser.
-
Brython: A Python 3 implementation for client-side web programming. It allows you to write Python code that runs in the browser, replacing JavaScript.
-
Pyodide: A Python distribution for the browser and Node.js based on WebAssembly. It allows you to run Python code in the browser and includes many popular scientific libraries.
-
Skulpt: A JavaScript implementation of Python. It allows you to run Python code in the browser without any server-side components.
These tools can be integrated into web pages to provide interactive Python code execution environments.
Setting Up
For the setup, in depends on your web environment. If your environment is plain JavaScript, you can use plain library such as runno that adds custom web element to your page. Using the custom element, it can run any python code (with some limitation depending on the interpreter).
One of the possible setup is React based web. In case of React, LiveCodes provide custom React component that allows embedding live coding editor.
The common steps are as follows:
Plan
- Install Dependencies: Ensure you have the necessary dependencies installed, including
livecodes
. - Import LiveCodes Component: Import the
LiveCodes
component from thelivecodes/react
package. - Define Options: Set up the options for the
LiveCodes
component, including the Python code to run and the configuration for the layout. - Use LiveCodes Component: Embed the
LiveCodes
component in your React App with the specified options.
Steps
-
Install Dependencies:
npm install livecodes
-
Import LiveCodes Component:
import LiveCodes from "livecodes/react";
-
Define Options:
export const options = { params: { python: "print('Hello, world!')", languages: "python", console: "full", compiled: "none", }, config: { layout: 'vertical', }, };
-
Use LiveCodes Component:
<LiveCodes client:load {...options} />
Running and Testing Python Code
Result:
Codes:
import LiveCodes from "livecodes/react";
export const options = {
params: {
python: "print('Hello, world!')",
languages: "python",
console: "full",
compiled: "none",
},
config: {
layout: 'vertical',
},
};
<LiveCodes client:load {...options} />
For simple testing without any installation, public hosted live code such as ReplIt can be used.
Here is an example of using LiveCodes in Basic React Template https://replit.com/@mohhasbias/React-Javascript#src/App.jsx
Conclusion
Numerous benefits are offered by embedding Python code in web pages, such as enabling interactive learning, providing real-time demonstrations, facilitating quick prototyping, and increasing user engagement. By using tools like Runno, LiveCodes, Brython, Pyodide, and Skulpt, Python code execution can be easily integrated into the web environment. Experimentation with these tools is encouraged to enhance web content and create more interactive and engaging experiences for users.